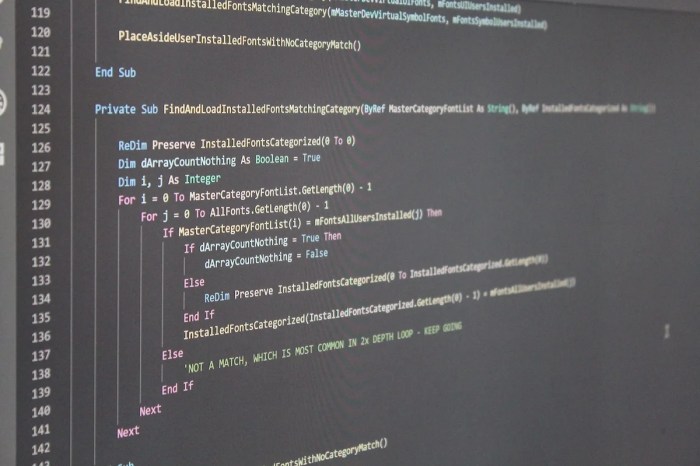
Handle Errors in Microsoft VBA: A Comprehensive Guide
Handle errors microsoft vba – Handle Errors in Microsoft VBA: A Comprehensive Guide is your guide to understanding and implementing robust error handling techniques within your VBA code. Imagine a world where your VBA applications gracefully handle unexpected issues, preventing crashes and providing informative feedback to users.
This guide will empower you to write error-resistant code, transforming your VBA projects from fragile to resilient.
Error handling is essential for creating reliable VBA applications. Unhandled errors can lead to crashes, data loss, and a frustrating user experience. This guide will equip you with the knowledge and skills to anticipate potential errors, gracefully handle them, and ensure the smooth operation of your VBA applications.
Introduction to Error Handling in VBA: Handle Errors Microsoft Vba
Error handling is a crucial aspect of writing robust and reliable VBA applications. It involves anticipating and gracefully managing errors that may occur during the execution of your code, preventing unexpected crashes and ensuring the stability of your application.
Importance of Error Handling
Effective error handling in VBA is essential for creating applications that are resilient to unforeseen issues. By anticipating potential errors and implementing appropriate mechanisms to handle them, you can enhance the reliability and user experience of your VBA applications.
Potential Consequences of Unhandled Errors
Unhandled errors in VBA can lead to various undesirable consequences, including:
Application Crashes
The most immediate and disruptive consequence of unhandled errors is the termination of your VBA application. This can result in data loss, incomplete tasks, and frustration for users.
Data Corruption
Debugging VBA code can be a real headache, especially when you encounter those pesky runtime errors. It’s like trying to keep your outdoor metal furniture looking pristine – you need the right tools and techniques to protect it from the elements.
Just like learning how to weatherproof your metal furniture for the outdoors can save you from rust and corrosion, understanding how to handle errors in VBA can prevent your code from crashing and ensure a smooth, robust application.
Errors can corrupt data, leading to inconsistencies and inaccuracies. This can have serious implications for the integrity and reliability of your application.
Unpredictable Behavior
Unhandled errors can cause unpredictable behavior, making it difficult to diagnose and fix issues. This can lead to confusion and frustration for users.
Handling errors in Microsoft VBA can be a real headache, especially when you’re trying to debug a complex macro. Sometimes, it’s good to take a break and remember that life is about more than just code. Check out this awesome article on sister style get happy for some inspiration and a fresh perspective.
Once you’ve recharged, you’ll be ready to tackle those pesky VBA errors with renewed vigor!
Security Risks
In some cases, unhandled errors can create security vulnerabilities, exposing your application to malicious attacks.
Common Errors Encountered in VBA Code
VBA applications can encounter various errors during their execution. Some common errors include:
Type Mismatch
Occurs when you attempt to assign a value of an incompatible data type to a variable. For example, assigning a string value to a variable declared as an integer.
Division by Zero
Occurs when you attempt to divide a number by zero.
Subscript Out of Range
Occurs when you attempt to access an element in an array that is beyond its valid range.
Object Not Found
Occurs when you try to access an object that does not exist.
File Not Found
Occurs when you try to open or access a file that does not exist.
Run-time Error 13
Type Mismatch: This is a very common error that can occur in various situations, such as when you try to assign a value of the wrong data type to a variable, or when you try to use an object that is not the correct type.
VBA Error Handling Techniques
VBA provides robust mechanisms to handle runtime errors, ensuring your code continues to execute even when unexpected situations arise. This section delves into the core techniques and strategies to gracefully manage errors in your VBA projects.
The On Error Statement
The `On Error` statement is the cornerstone of VBA error handling. It defines the actions to be taken when an error occurs within a specific code block.The `On Error` statement has several options:
- On Error Resume Next:This option instructs VBA to ignore the current error and continue execution with the next line of code. This approach can be useful when you expect certain errors to occur and want to handle them manually. However, it can also mask critical errors, making it difficult to debug your code.
- On Error GoTo 0:This option disables error handling for the current code block, forcing VBA to halt execution and display an error message to the user. This is useful for testing purposes or when you want to explicitly handle errors in a specific way.
- On Error GoTo [Label]:This option redirects execution to a specific line of code marked with a label. This allows you to handle errors in a dedicated error-handling routine.
The Err Object, Handle errors microsoft vba
The `Err` object provides detailed information about the error that has occurred. Its properties are crucial for understanding and handling errors effectively.
- Err.Number:This property returns the error code, which is a unique numerical identifier for the specific error that occurred. This code can be used to identify and handle specific errors.
- Err.Description:This property returns a descriptive text string explaining the error that occurred. This description is helpful for understanding the nature of the error and for providing informative error messages to the user.
- Err.Source:This property identifies the module or object where the error occurred. This information is useful for pinpointing the location of the error and for debugging purposes.
- Err.HelpFile:This property specifies the help file that contains information about the error. This can be helpful for getting more details about the error and its potential causes.
- Err.HelpContext:This property provides the context ID within the help file that contains information about the error. This helps you navigate to the relevant section of the help file.
Error Handling Techniques
VBA offers various approaches to handling errors. The choice of approach depends on the specific needs of your code and the level of error control you require.
- Using the On Error Statement:This is the traditional approach to error handling in VBA. It allows you to control the flow of execution when an error occurs. However, it can be less structured and may require careful attention to error handling logic.
- Using Try…Catch Blocks:Introduced in VBA 7.0 (Office 2003), Try…Catch blocks provide a more structured and readable way to handle errors. This approach is particularly beneficial for complex code blocks where multiple errors might occur.
Try[Code that may raise errors] Catch [Error Type] [Error Variable][Error handling code for the specific error type] End Try
This block executes the code within the `Try` section. If an error occurs, the corresponding `Catch` block will handle the error. You can define multiple `Catch` blocks to handle different error types.
Common Error Handling Scenarios
Error handling is essential in VBA to prevent unexpected program crashes and ensure smooth operation. Common scenarios require specific error handling routines to address potential issues and maintain program stability.
File I/O Operations
File I/O operations, like reading or writing to files, can encounter various errors. For example, the file might not exist, be inaccessible, or have insufficient permissions. Error handling routines should be designed to anticipate these situations and take appropriate actions.
For instance, if a file cannot be opened for reading, the code can prompt the user to provide a valid file path or display an error message. Here are some common errors encountered during file I/O operations:* File not found:This error occurs when the specified file does not exist.
Permission denied
The user might lack the necessary permissions to access the file.
Disk full
The disk drive might be full, preventing the file from being written.
File in use
The file might be open in another application, preventing access.
Example:
Sub OpenFile() On Error GoTo FileError Dim strFileName As String strFileName = "C:\My Documents\MyFile.txt" Open strFileName For Input As #1 ' Process the file contents Close #1 Exit SubFileError: MsgBox "Error opening file: " & Err.DescriptionEnd Sub
Database Interactions
Database interactions involve connecting to a database, executing queries, and retrieving or updating data. Errors can occur during any of these steps. For instance, the database might be unavailable, the query might be invalid, or there might be data integrity issues.
Error handling routines for database interactions should be designed to handle these potential problems and ensure data consistency. For example, if a database connection fails, the code can retry the connection or display an error message to the user.Common errors encountered during database interactions include:* Connection failed:The database server might be unavailable or the connection credentials might be incorrect.
Query error
The SQL query might be invalid or contain syntax errors.
Data integrity error
The data being inserted or updated might violate database constraints.
Transaction error
A database transaction might fail due to various reasons, such as insufficient permissions or data integrity violations.
Example:
Sub UpdateDatabase() On Error GoTo DatabaseError Dim strSQL As String strSQL = "UPDATE Customers SET Name = 'John Doe' WHERE ID = 1" ' Execute the SQL query ' Handle the results Exit SubDatabaseError: MsgBox "Error updating database: " & Err.DescriptionEnd Sub
Network Communication
Network communication involves sending and receiving data over a network. Errors can occur during network communication due to network connectivity issues, server problems, or data transmission errors.Error handling routines for network communication should be designed to handle these situations gracefully and ensure data integrity.
For example, if a network connection is lost, the code can retry the connection or display an error message to the user.Common errors encountered during network communication include:* Connection timeout:The network connection might take too long to establish.
Server error
The server might be unavailable or experiencing technical difficulties.
Data transmission error
Handling errors in Microsoft VBA can be a real pain, especially when you’re trying to build something complex. It’s like trying to design a new home without a mood board – you’re just throwing things together hoping they’ll work.
But with a clear plan, like Laura’s inspiring new home mood board , you can approach your VBA error handling with more confidence. By anticipating potential problems and implementing robust error traps, you can create a much more stable and reliable codebase.
The data being transmitted might be corrupted or incomplete.
Network access denied
The user might lack the necessary permissions to access the network resource.
Example:
Sub SendData() On Error GoTo NetworkError Dim strURL As String strURL = "http://www.example.com/data.txt" ' Send data to the server ' Handle the response Exit SubNetworkError: MsgBox "Error sending data: " & Err.DescriptionEnd Sub
User Input Validation
User input validation is crucial to ensure that the data entered by the user is valid and meets the program’s requirements. Errors can occur if the user enters invalid data, such as incorrect data types, invalid formats, or out-of-range values.Error handling routines for user input validation should be designed to prevent these errors and guide the user to enter valid data.
For example, if the user enters an invalid date format, the code can display an error message and prompt the user to enter a valid date.Common errors encountered during user input validation include:* Invalid data type:The user might enter a value that is not of the expected data type.
Invalid format
The user might enter a value that does not adhere to the required format.
Out-of-range value
The user might enter a value that falls outside the allowed range.
Duplicate entry
The user might enter a value that already exists in the system.
Example:
Sub ValidateInput() On Error GoTo InputError Dim strInput As String strInput = InputBox("Enter your age:") If IsNumeric(strInput) Then If CInt(strInput) >= 18 Then ' Valid input Else MsgBox "You must be at least 18 years old." Exit Sub End If Else MsgBox "Please enter a valid number." Exit Sub End If Exit SubInputError: MsgBox "Invalid input: " & Err.DescriptionEnd Sub
Advanced Error Handling Concepts
So far, we’ve covered the basics of error handling in VBA. Now, let’s delve into some advanced concepts that will empower you to write more robust and resilient code. These techniques will help you not only catch errors but also understand their root cause, making debugging and troubleshooting a breeze.
Debugging and Troubleshooting VBA Errors
Debugging and troubleshooting are essential skills for any VBA developer. While error handling helps you gracefully handle unexpected situations, understanding the root cause of errors is crucial for preventing them in the future. Let’s explore some techniques to help you identify and resolve errors effectively.
- Using the Immediate Window:The Immediate Window (Ctrl+G) is a powerful tool for debugging. You can use it to execute code snippets, print variable values, and even test specific lines of code.
- Breakpoints:Breakpoints allow you to pause code execution at specific lines. This lets you inspect variables, examine the program’s state, and step through code line by line to pinpoint the source of the error.
- Step-by-Step Execution:The “Step Into” (F8) and “Step Over” (Shift+F8) features allow you to execute code line by line, giving you granular control over the execution flow.
- Using the Locals Window:The Locals Window displays the values of variables within the current scope. This helps you understand the data flow and identify potential issues with variable assignments or calculations.
- Error Object Properties:The Error object provides detailed information about the error that occurred. Properties like Number, Description, and Sourceoffer valuable insights into the nature of the error and its origin.
- Logging Errors:Implement logging to record error details, including the error number, description, timestamp, and other relevant information. This helps you track errors over time, identify recurring issues, and analyze patterns.
Best Practices for Robust Error Handling
Writing robust error handling code is crucial for building reliable VBA applications. By following these best practices, you can create code that gracefully handles errors, provides meaningful feedback to users, and prevents unexpected crashes.
- Specific Error Handling:Instead of using a generic On Error Resume Next, handle specific errors by using Err.Numberto identify the type of error. This allows you to take appropriate actions based on the specific error encountered.
- Informative Error Messages:Display clear and concise error messages to the user, explaining the problem and suggesting possible solutions. Avoid generic messages like “Error occurred” and provide specific details about the error.
- Error Logging:Log errors to a file or database for later analysis. This allows you to track errors, identify trends, and troubleshoot issues more effectively.
- Error Recovery:Implement strategies to recover from errors when possible. For example, if a file cannot be opened, you could prompt the user to select a different file or attempt to create the file.
- Clean Up Resources:Release any resources, such as file handles or database connections, before exiting an error handler. This prevents resource leaks and ensures the stability of your application.
Custom Error Handling Functions and Procedures
Custom error handling functions and procedures allow you to encapsulate error handling logic and reuse it across your code. This promotes code reusability and consistency.
- Create a Centralized Error Handling Routine:Define a dedicated function or procedure to handle errors centrally. This function can log errors, display user-friendly messages, and perform other necessary actions.
- Pass Error Information:When calling the error handling routine, pass the error number, description, and any other relevant information. This allows the routine to provide context-specific error messages and actions.
- Use Error Codes and Descriptions:Define a set of error codes and corresponding descriptions to standardize error reporting. This makes it easier to understand and troubleshoot errors.
- Implement Custom Error Classes:For more complex error handling scenarios, consider creating custom error classes. This allows you to define specific error types and properties, providing greater control over error handling.
VBA Error Codes and Descriptions
VBA defines a set of standard error codes that provide information about the type of error that occurred. Understanding these codes can help you identify and resolve errors more efficiently. Here’s a table listing some common VBA error codes, their descriptions, recommended actions, and example code snippets:
Error Code | Error Description | Recommended Action | Example Code Snippet |
---|---|---|---|
13 | Type mismatch | Check the data types of the variables involved in the operation. Ensure that the data types are compatible. | Dim myVar As IntegermyVar = "Hello" ' This will cause a type mismatch error |
1004 | Application-defined or object-defined error | This error usually indicates a problem with the specific application or object you’re trying to use. Check the documentation for the application or object for more information. | ActiveWorkbook.SaveAs "C:\MyFile.xls" ' This may cause a 1004 error if the user does not have write permissions to the specified directory. |
9 | Subscript out of range | Ensure that the array index or object property you’re accessing is within the valid range. | Dim myArray(10) As IntegermyArray(11) = 10 ' This will cause a subscript out of range error |
424 | Object required | This error occurs when you try to access a property or method of an object that hasn’t been properly defined or initialized. | Dim mySheet As WorksheetmySheet.Range("A1").Value = "Hello" ' This will cause an object required error if mySheet has not been properly set to a worksheet object. |
5 | Invalid procedure call or argument | Check the arguments you’re passing to the function or procedure. Ensure that the number and type of arguments are correct. | Dim myVar As IntegermyVar = Application.WorksheetFunction.Sum("A1", "B1") ' This will cause an invalid procedure call or argument error because the Sum function expects a range as an argument. |
Error Logging and Reporting
Error logging and reporting are crucial aspects of effective error handling in VBA. They provide valuable insights into the causes of errors, enabling developers to debug, troubleshoot, and resolve issues efficiently. By systematically recording error information, you can track down the root cause of problems, identify recurring patterns, and improve the overall stability and reliability of your VBA applications.
Methods for Recording Errors
Recording errors involves capturing relevant details about the error, such as the error code, error description, date and time of occurrence, and any additional context that might be helpful for debugging. You can implement error logging using various methods, including:
- Log Files:A simple and common approach is to write error information to a text file. You can use the `FileSystemObject` to create and write to a log file.
- Databases:For more structured and persistent logging, you can store error details in a database table. This allows for querying and analysis of error data.
- Event Log:Windows provides an event log service where you can record application events, including errors. This can be useful for centralized error management.
Designing a Simple Error Reporting System
A basic error reporting system can be designed using VBA to capture and report error details to a designated location. This system typically involves the following steps:
- Error Handling Routine:Implement an error handling routine that intercepts errors using the `On Error` statement.
- Error Information Gathering:Within the error handling routine, capture relevant error information, such as the error number, description, and any additional context.
- Error Reporting Mechanism:Choose a method to report the error information, such as writing to a log file, sending an email, or displaying a message box.
- Error Logging:Record the error information in a chosen format, such as a text file or a database.
Example:“`vbaSub MyProcedure() On Error GoTo HandleError ‘ … Code that might generate errors …HandleError: ‘ Capture error information Dim errNum As Long, errDesc As String errNum = Err.Number errDesc = Err.Description ‘ Log the error to a file Dim fso As Object, logFile As Object Set fso = CreateObject(“Scripting.FileSystemObject”) Set logFile = fso.OpenTextFile(“C:\ErrorLog.txt”, 8, True) logFile.WriteLine “Error occurred on: ” & Date & ” at ” & Time logFile.WriteLine “Error Number: ” & errNum logFile.WriteLine “Error Description: ” & errDesc logFile.Close ‘ Display an error message MsgBox “An error occurred.
See the error log for details.” ‘ Resume execution or exit Resume Next ‘ Continue execution ‘ Exit Sub ‘ Terminate the procedureEnd Sub“`